In this example we will be using Netbeans as an IDE and hibernate,spring & jsf framework which provides a very good platform for building enterprise application & the steps are as follows:
1)
Create a table named “tbl_Departments” in mysql databaseCREATE TABLE `tbl_departments` (
`DepartmentID` int(10) NOT NULL auto_increment,
`Title` varchar(256) NOT NULL,
`Description` varchar(256),
PRIMARY KEY (`DepartmentID`)
);
2) Create a “Department” entity class from the netbeansusing “New->Other->Persistence->Entity classes from Database” package com.models;
@Entity @Table(name = "tbl_departments") public class Department implements Serializable { private static final long serialVersionUID = 1L;
@Id @GeneratedValue(strategy=GenerationType.IDENTITY) @Column(name = "DepartmentID", nullable = false) private Integer departmentID; @Column(name = "Title", nullable = false) private String title; @Column(name = "Description") private String description; } |
3)
Create a DAO interface named “DepartmentDAO”
package com.dao;
import com.models.Department; import java.util.List; import org.springframework.transaction.annotation.Transactional;
public interface DepartmentDAO { @Transactional(readOnly=false) void deleteDepartment(Department dep);
@Transactional(readOnly=false) List<Department> getDepartments();
@Transactional(readOnly=false) void insertDepartment(Department dep); @Transactional(readOnly=false) void updateDepartment(Department dep); @Transactional(readOnly=false) Department getDepartmentByID(int departmentID); } |
4) Create a DAO implementation class named “DepartmentDAOImpl” extending the “HibernateDaoSupport”
class provided by the Spring & implementing “DepartmentDAO” interface. Using the spring dao, we can minimize the code required for hibernate to interact with the database.
package com.dao.impl;
import com.dao.DepartmentDAO; import com.models.Department; import java.util.List; import org.springframework.orm.hibernate3.support.HibernateDaoSupport;
public class DepartmentDAOImpl extends HibernateDaoSupport implements DepartmentDAO { public void deleteDepartment(Department dep) { getHibernateTemplate().delete(dep); } public Department getDepartmentByID(int departmentID) { return (Department) getHibernateTemplate().load(Department.class, departmentID); } public List<Department> getDepartments() { return (List<Department>) getHibernateTemplate().find("from Department d order by d.title "); } public void insertDepartment(final Department dep) { getHibernateTemplate().save(dep); } public void updateDepartment(Department dep) { getHibernateTemplate().update(dep); } } |
5)
Create spring bean configuration file named “beans.xml”
<beans> <bean id="sessionFactory" class="org.springframework.orm.hibernate3.annotation.AnnotationSessionFactoryBean"> <property name="annotatedClasses"> <list> <value>com.models.Department</value> </list> </property> <property name="hibernateProperties"> <props> <prop key="hibernate.show_sql">true </prop> <prop key="hibernate.transaction.factory_class"> org.hibernate.transaction.JDBCTransactionFactory </prop> <prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialect </prop> <prop key="hibernate.connection.driver_class">com.mysql.jdbc.Driver </prop> <prop key="hibernate.connection.url">jdbc:mysql://localhost/shoppro </prop> <prop key="hibernate.connection.username">root </prop> <prop key="hibernate.connection.password">root </prop> </props> </property> </bean> <tx:annotation-driven transaction-manager="transactionManager"/>
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory"> <ref local="sessionFactory"/> </property> </bean> <bean class="org.springframework.beans.factory.annotation.RequiredAnnotationBeanPostProcessor"/>
<!-- Define our Data Access beans --> <bean id="departmentDao" class="com.dao.impl.DepartmentDAOImpl"> <property name="sessionFactory" ref="sessionFactory"/> </bean> </beans> |
6)
Now create a backing bean class named “ManageDepartments” for jsf
package com.managedbeans;
public class ManageDepartments {
private String departmentID; private String title; private String description;
public ManageDepartments() { dc = Helper.getDepartmentDAO(); departments = dc.getDepartments(); }
private UIDataTable table; private List<Department> departments; private DepartmentDAO dc ; private HtmlAjaxCommandLink updateLink; private boolean editable = false; //editable mode means editable or not public void editAction(ActionEvent e) { Department dep = (Department) table.getRowData();
this.setDepartmentID(String.valueOf(dep.getDepartmentID())); //save into table as attribute also for postback retrieval table.getAttributes().put("depID",dep.getDepartmentID() );
this.setTitle(dep.getTitle()); this.setDescription(dep.getDescription());
this.setEditable(true); this.updateLink.setRendered(true); }
public void updateAction(ActionEvent e) { Department dep = new Department();
dep.setDepartmentID( (Integer)table.getAttributes().get("depID")); dep.setTitle(this.getTitle()); dep.setDescription(this.getDescription());
dc.updateDepartment(dep); this.clearFields(); this.setEditable(false); this.updateLink.setRendered(false); this.departments = dc.getDepartments(); }
public void cancelAction(ActionEvent e){ this.clearFields(); this.setEditable(false); this.updateLink.setRendered(false); }
public void deleteAction(ActionEvent e) { if (table.getRowData() != null) { Department dep = (Department) table.getRowData(); dc.deleteDepartment(dep); this.clearFields(); updateLink.setRendered(false); this.departments = dc.getDepartments(); } }
public void insertAction(ActionEvent e) { Department dep = new Department(); dep.setDepartmentID(0); dep.setTitle(this.getTitle()); dep.setDescription(this.getDescription()); dc.insertDepartment(dep);
this.clearFields(); this.departments = dc.getDepartments(); }
void clearFields() { this.setTitle(""); this.setDescription(""); this.setDepartmentID(""); }
}
|
7) Create and configure “faces-config.xml” & “web.xml” for jsf & managed bean
<faces-config> <managed-bean> <managed-bean-name>ManageDepartments</managed-bean-name> <managed-bean-class>com.managedbeans.ManageDepartments</managed-bean-class> <managed-bean-scope>request</managed-bean-scope> </managed-bean> </faces-config> |
8)
At last create a jsp page containing jsf & richfaces tags named “manageDepartments.jsp”
<%@taglib prefix="f" uri="http://java.sun.com/jsf/core"%> <%@taglib prefix="h" uri="http://java.sun.com/jsf/html"%>
<%@ taglib uri="http://richfaces.org/a4j" prefix="a4j"%> <%@ taglib uri="http://richfaces.org/rich" prefix="rich"%>
<html> <body> <f:view> <h:form id="frm"> <rich:panel header="Deparments" style="width:450px"> <rich:dataTable binding="#{ManageDepartments.table}" onRowMouseOver="this.style.backgroundColor='#B5CEFD'" onRowMouseOut="this.style.backgroundColor='#{org.richfaces.SKIN.tableBackgroundColor}'" onRowClick="this.style.backgroundColor='#F1F1F1'" rows="10" width="100%" id="tbl" value="#{ManageDepartments.departments}" var="d"> <rich:column> <f:facet name="header"> <h:outputText value="Title" /> </f:facet> <h:outputText value="#{d.title}" /> </rich:column> <rich:column width="230px"> <f:facet name="header"> <h:outputText value="Description" /> </f:facet> <h:outputText value="#{d.description}" /> </rich:column> <rich:column style="text-align:center"> <f:facet name="header"> <h:outputText value="Edit" /> </f:facet> <a4j:commandLink reRender="pnl" actionListener="#{ManageDepartments.editAction}"> <h:graphicImage style="border:0"url="/images/Edit.gif" /> </a4j:commandLink> </rich:column> <rich:column style="text-align:center"> <f:facet name="header"> <h:outputText value="Delete" /> </f:facet> <a4j:commandLink reRender="tbl,pnl" actionListener="#{ManageDepartments.deleteAction}" onclick="if(confirm('Confirm delete?') == false ) return false;" > <h:graphicImage style="border:0" url="/images/Delete.gif" /> </a4j:commandLink> </rich:column> <f:facet name="footer"> <rich:datascroller /> </f:facet>
</rich:dataTable> </rich:panel> <br> <rich:panel header="Department" style="width:450px"> <h:panelGrid id="pnl" columns="2" width="100%" >
<h:outputText value="Department ID:" rendered="#{ManageDepartments.editable}" /> <h:outputText value="#{ManageDepartments.departmentID}" rendered="#{ManageDepartments.editable}" />
<h:outputText value="Title:" /> <h:panelGroup> <h:inputText id="title" value="#{ManageDepartments.title}" style="width:100%" /> <h:message style="color:red" for="title" /> </h:panelGroup>
<h:outputText value="Description:" /> <h:inputTextarea style="width:100%" value="#{ManageDepartments.description}" />
<a4j:commandLink value="Insert" reRender="tbl,pnl" actionListener="#{ManageDepartments.insertAction}" rendered="#{not ManageDepartments.editable}"/> <a4j:commandLink value="Update" reRender="tbl,pnl" actionListener="#{ManageDepartments.updateAction}" rendered="false" binding="#{ManageDepartments.updateLink}" /> <a4j:commandLink value="Cancel" reRender="pnl,tbl" actionListener="#{ManageDepartments.cancelAction}" /> </h:panelGrid> </rich:panel> </h:form> </f:view> </body> </html>
|
Outputs
Case 1: displaying departments details in the dataTable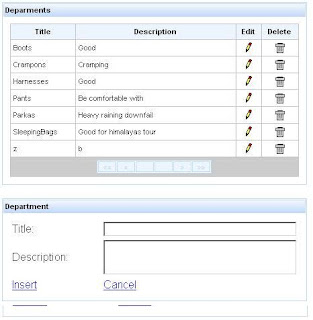
Case2: when the user selects a department for editing, its information appeared in the inputText & outputText with update & cancel buttons
Source Code with out libraries